Javascript Algorithms And Data Structures
TOPIC
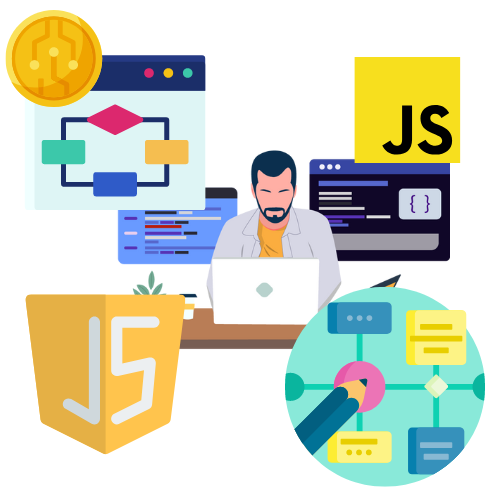
Javascript Algorithms And Data Structures
JavaScript is a high-level programming language that all modern web browsers support. It is also one of the core technologies of the website with HTML and CSS, which you may have learned before. This section will cover basic JavaScript programming concepts ranging from variables and arithmetic to objects and loops.
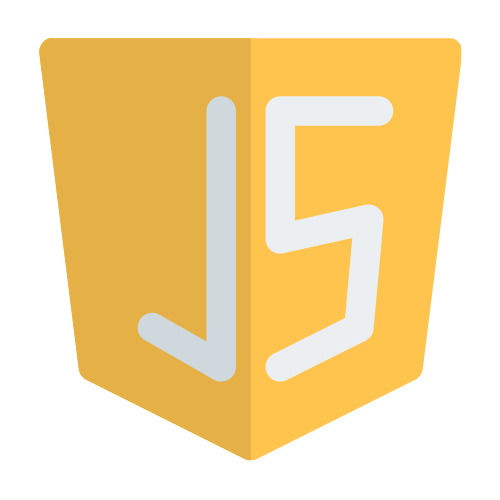
Basic JavaScript
Introduction to Basic JavaScript
JavaScript is a versatile and widely used programming language that allows developers to add interactivity, dynamic content, and functionality to websites and web applications. As one of the core technologies of the web, JavaScript enables web pages to respond to user actions, manipulate data, and modify the content of a webpage on the fly without requiring a page reload. It is essential for front-end web development and increasingly used on the server-side through platforms like Node.js.
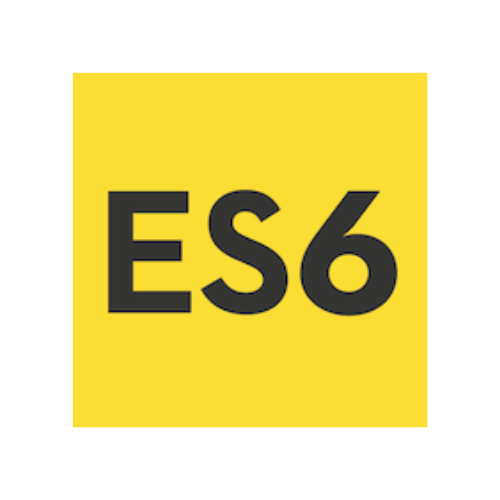
ES6
Introduction to ES6
ES6 (ECMAScript 2015) is the sixth edition of the ECMAScript standard, which is the scripting language specification that JavaScript is based on. ES6 introduced significant enhancements and new features to the JavaScript language, making it more powerful, efficient, and developer-friendly. These improvements have become the foundation for modern JavaScript development.
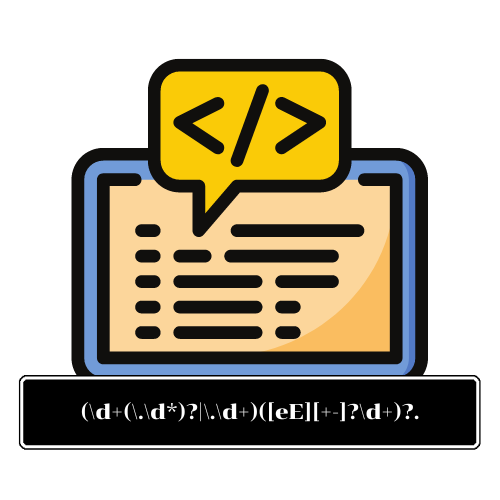
Regular Expressions
Introduction to Regular Expressions
Regular Expressions, often referred to as regex or regexp, are powerful and versatile patterns used to search, match, and manipulate text in strings. They are a fundamental tool in text processing, providing a concise and flexible way to describe complex search patterns. Regular expressions are supported in many programming languages, text editors, and command-line tools.
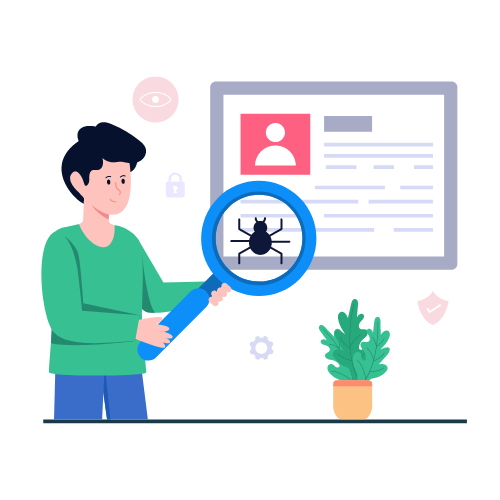
Debugging
Introduction to Debugging
Debugging is the process of identifying and fixing errors, bugs, or issues in software code. It is an essential skill for developers as it helps ensure that programs run smoothly and as intended. Debugging involves investigating the code to understand the root cause of unexpected behavior and making necessary adjustments to correct the problem.
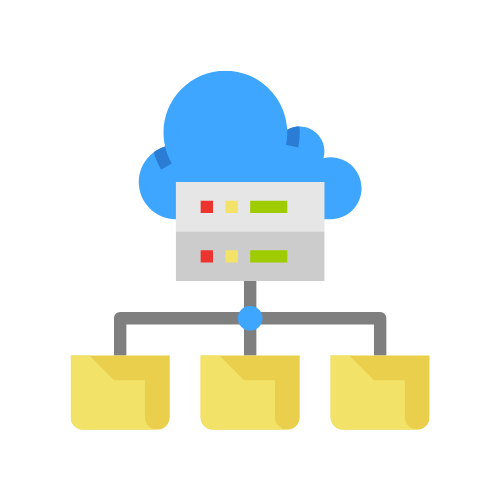
Basic Data Structures
Introduction to Basic Data Structures
Data structures are fundamental concepts in computer science and programming that allow developers to organize and store data efficiently. They provide a way to represent and manipulate data in a structured manner, enabling faster access, retrieval, and modification of information. Basic data structures are essential building blocks used in various algorithms and programs.
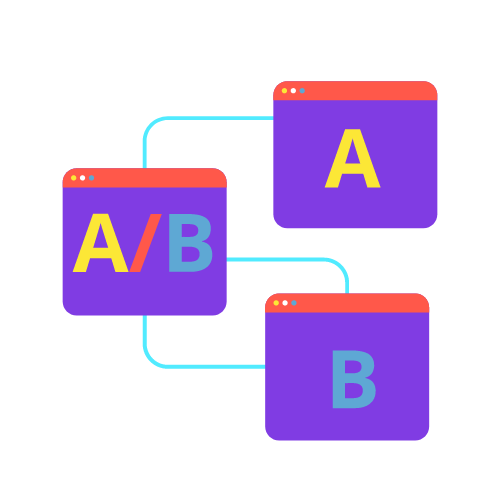
Basic Algorithm Scripting
Introduction to Basic Algorithm Scripting
Basic algorithm scripting refers to the process of solving simple coding challenges and problems using algorithms in programming. It is an essential part of learning to code and becoming a proficient programmer. Algorithm scripting helps developers improve problem-solving skills and understand fundamental programming concepts.
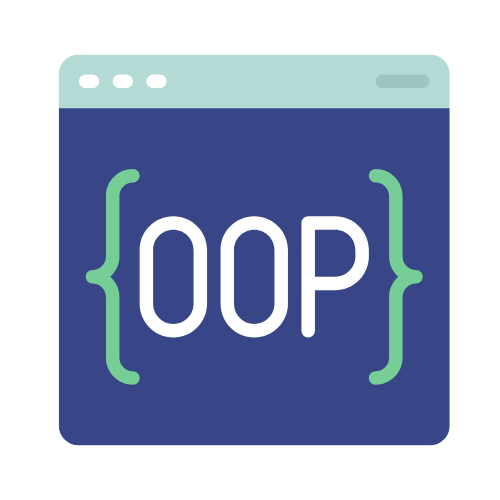
Object Oriented Programming
Introduction to Object Oriented Programming
CSS Grid is a layout module introduced in CSS3 that provides a powerful and flexible way to create two-dimensional grid-based layouts for web pages. Unlike CSS Flexbox, which focuses on arranging elements in a single dimension (either as rows or columns), CSS Grid allows designers and developers to define both rows and columns, offering precise control over the placement and sizing of elements within the grid.
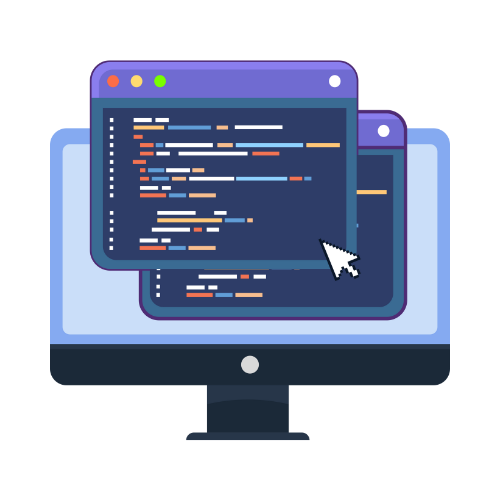
Functional Programming
Introduction to Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. In functional programming, the emphasis is on using functions to perform operations on data, rather than altering data directly through assignment and loops, as in imperative programming.
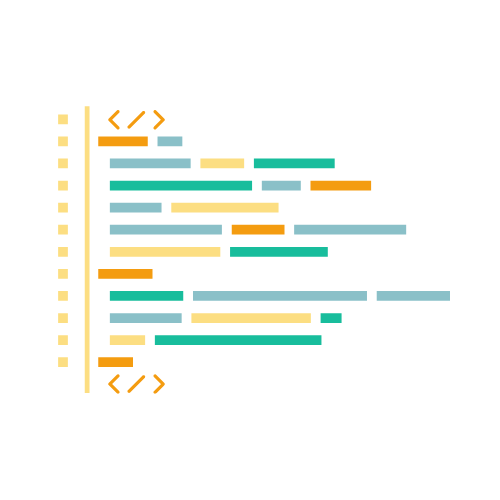
Intermediate Algorithm Scripting
Introduction to Intermediate Algorithm Scripting
Intermediate algorithm scripting refers to the process of solving more complex coding challenges and problems using algorithms in programming. It builds upon the concepts learned in basic algorithm scripting and involves more sophisticated problem-solving techniques and data manipulation.
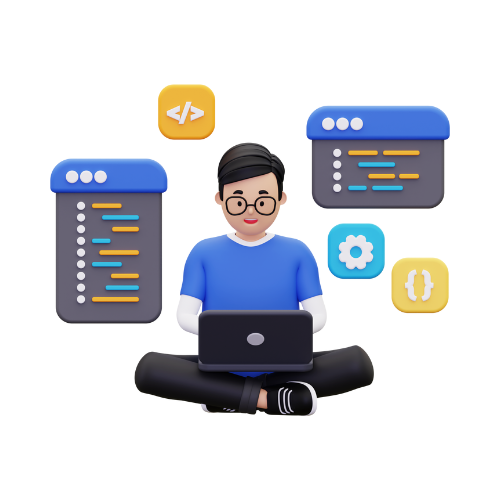
JavaScript Algorithms and Data Structures Projects
JavaScript Algorithms and Data Structures Projects
JavaScript Algorithms and Data Structures Projects refer to a set of coding challenges and projects that focus on implementing various algorithms and data structures using JavaScript. These projects are designed to help developers improve their problem-solving skills, practice algorithmic thinking, and gain hands-on experience in working with data structures.